OBD设备
在此之前,从未接触OBD这个东西,蓝牙也少有接触,谨以此篇来记录一下与OBD设备通信的开发过程
申请权限
首先在清单文件中添加蓝牙权限
1 2 3 4
| <uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
|
打开蓝牙
创建一个OBDManager
类,添加一个getInstance()
方法,使用单例模式获取当前类的实例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public class OBDManager { private static OBDManager obdManager;
private OBDManager() {}
public static OBDManager getInstance() { if (obdManager == null) { obdManager = new OBDManager(); } return obdManager; } }
|
添加一个init()
方法,为该类提供简单的初始化
1 2 3 4 5 6 7 8 9 10 11 12
| private BluetoothAdapter bluetoothAdapter;
public void init() { bluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); }
|
判断设备是否拥有蓝牙功能,Android模拟器是提供不了蓝牙功能的
1 2 3 4 5 6
|
public boolean isHasBluetooth(Context context) { return (bluetoothAdapter != null && context.getPackageManager().hasSystemFeature(PackageManager.FEATURE_BLUETOOTH)); }
|
判断当前是否已经打开蓝牙,打开\关闭蓝牙
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
|
public boolean isEnableBluetooth() { return bluetoothAdapter.isEnabled(); }
public boolean enableBluetooth() { return bluetoothAdapter.enable(); }
public void enableBluetooth(Activity activity) { Intent intent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); activity.startActivityForResult(intent, 1); }
public boolean disableBluetooth() { return bluetoothAdapter.disable(); }
|
扫描蓝牙
在真正开始搜索蓝牙设备之前,需要先注册一个广播,在广播中接收系统搜索到的蓝牙设备,并统一添加到一个集合中,然后添加一个回调事件,用以再扫描到设备时调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95
|
public void register(Context context) { IntentFilter intentFilter = new IntentFilter(); intentFilter.addAction(BluetoothDevice.ACTION_FOUND); intentFilter.addAction(BluetoothDevice.ACTION_BOND_STATE_CHANGED); context.registerReceiver(bluetoothReceiver, intentFilter); }
public void unregister(Context context) { context.unregisterReceiver(bluetoothReceiver); }
private final List<BluetoothDevice> bluetoothDeviceList = new ArrayList<>();
public List<BluetoothDevice> getBluetoothDeviceList() { return bluetoothDeviceList; }
public void clearBluetoothDeviceList() { bluetoothDeviceList.clear(); }
private final BroadcastReceiver bluetoothReceiver = new BroadcastReceiver() { @Override public void onReceive(Context context, Intent intent) { String action = intent.getAction(); if (action != null) { if (action.equals(BluetoothDevice.ACTION_FOUND)) { BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE); if (device.getName() != null && device.getAddress() != null && !isExistDevice(device)) { bluetoothDeviceList.add(device); if (onScanBluetoothDeviceListener != null) { onScanBluetoothDeviceListener.OnScan(device); } } } else if (action.equals(BluetoothDevice.ACTION_BOND_STATE_CHANGED)) { int state = intent.getIntExtra(BluetoothDevice.EXTRA_BOND_STATE,BluetoothDevice.ERROR); int prevState = intent.getIntExtra(BluetoothDevice.EXTRA_PREVIOUS_BOND_STATE,BluetoothDeviceERROR); if (state == BluetoothDevice.BOND_BONDED && prevState == BluetoothDevice.BOND_BONDING) { } else if (state == BluetoothDevice.BOND_BONDING && prevState == BluetoothDevice.BOND_NONE) { } else if (state == BluetoothDevice.BOND_NONE) { } } } }
private boolean isExistDevice(BluetoothDevice newDevice) { for (BluetoothDevice bluetoothDevice : bluetoothDeviceList) { if (bluetoothDevice.getAddress().equals(newDevice.getAddress())) { return true; } } return false; } };
private OnScanBluetoothDeviceListener onScanBluetoothDeviceListener;
public interface OnScanBluetoothDeviceListener{ void OnScan(BluetoothDevice bluetoothDevice); }
|
开始搜索蓝牙设备,停止搜索蓝牙设备,在开始搜索蓝牙设备的方法中传入回调方法,用以在搜索到蓝牙设备时调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
|
public synchronized void startScanBluetoothDevices(OnScanBluetoothDeviceListener onScanBluetoothDeviceListener) { if (!isEnableBluetooth()) { enableBluetooth(); }
this.onScanBluetoothDeviceListener = onScanBluetoothDeviceListener;
bluetoothAdapter.startDiscovery(); }
public void cancelScanBluetoothDevices() { if (!isEnableBluetooth()) { return; } bluetoothAdapter.cancelDiscovery(); }
|
配对蓝牙
调用setPin()
方法使系统自动输入配对码,免去用户手动输入的繁琐,调用setPairingConfirmation()
方法设置是否确认当前的配对,免去用户手动点击确认配对,调用createBond()
即开始进行蓝牙配对
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
|
public boolean createBond(BluetoothDevice bluetoothDevice) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) { return bluetoothDevice.createBond(); } else { try { Method method = bluetoothDevice.getClass().getMethod("createBond"); return (boolean) method.invoke(bluetoothDevice); } catch (Exception e) { e.printStackTrace(); } } return false; }
public boolean setPairingConfirmation(BluetoothDevice bluetoothDevice, boolean confirm) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) { return bluetoothDevice.setPairingConfirmation(confirm); } else { try { Method method = bluetoothDevice.getClass().getMethod("setPairingConfirmation", boolean.class); return (boolean) method.invoke(bluetoothDevice, confirm); } catch (Exception e) { e.printStackTrace(); } } return false; }
public boolean setPin(BluetoothDevice bluetoothDevice, byte[] pin) { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) { return bluetoothDevice.setPin(pin); } else { try { Method method = bluetoothDevice.getClass().getMethod("setPin", byte[].class); return (boolean)method.invoke(bluetoothDevice, new Object[]{pin}); } catch (Exception e) { e.printStackTrace(); } } return false; }
|
然后写一个bondDevice()方法来调用这三个方法,注意调用顺序:setPin()
、setPairingConfirmation()
、createBond()
,这里需要注意的是,我所开发的软件是一个拥有所有权限的系统软件,所以可以不经过用户操作自动配对蓝牙设备,正常的三方软件开发过程中,setPairingConfirmation()
会发生异常,在删除该方法的调用后,系统会显示一个允许配对的对话框
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
public void bondDevice(BluetoothDevice bluetoothDevice, String pinCode) { if (!TextUtils.isEmpty(pinCode)) { setPin(bluetoothDevice, pinCode.getBytes()); } setPairingConfirmation(bluetoothDevice, true); boolean bond = createBond(bluetoothDevice); if (bond) { } else { } }
public boolean isBondDevice(BluetoothDevice bluetoothDevice) { return bluetoothDevice.getBondState() == BluetoothDevice.BOND_BONDED; }
|
连接蓝牙
经典蓝牙的连接相当于在两个设备之间建立了socket连接,是个耗时操作,所以需要在子线程中执行
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
|
private class ConnectThread extends Thread {
private final BluetoothSocket bluetoothSocket;
public ConnectThread(BluetoothSocket socket) { bluetoothSocket = socket; }
@Override public void run() { super.run(); cancelScanBluetoothDevices(); try { bluetoothSocket.connect(); } catch (IOException e) { e.printStackTrace(); closeConnect(); } }
public void closeConnect() { if (bluetoothSocket != null) { try { bluetoothSocket.close(); } catch (IOException e) { e.printStackTrace(); } } } }
|
使用一个connectToDevice()
方法连接设备,传入的bluetoothDevice
所代表的设备一定要已经成功配对,注意这里用到的UUID,它是一个预定的建立与OBD之间的连接所需的UUID,另外注意我们要使用createRfcommSocketToServiceRecord将本机作为一个客户端建立与OBD之间的连接,因为OBD是一种蓝牙设备,是一个服务器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| private final UUID uuid_obd = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB"); private ConnectThread connectThread;
public synchronized void connectToDevice(BluetoothDevice bluetoothDevice) { try { BluetoothSocket socket = bluetoothDevice.createRfcommSocketToServiceRecord(uuid_obd); if (connectThread != null) { connectThread.closeConnect(); connectThread = null; } connectThread = new ConnectThread(socket); connectThread.start(); } catch (IOException e) { e.printStackTrace(); } }
|
蓝牙通信
创建一个MessageThread
类,在这个类中完成两个蓝牙设备之间的通信
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86
| private MessageThread messageThread; private String message;
private class MessageThread extends Thread {
private final InputStream inputStream; private final OutputStream outputStream; private boolean isReceiveMsg = false;
public MessageThread (BluetoothSocket socket) { InputStream _input = null; OutputStream _output = null;
try { _input = socket.getInputStream(); _output = socket.getOutputStream(); } catch (IOException e) { e.printStackTrace(); }
inputStream = _input; outputStream = _output; }
@Override public void run() { super.run(); byte[] buffer = new byte[1024]; int numBytes; isReceiveMsg = true;
while (isReceiveMsg) { try { numBytes = inputStream.read(buffer); if (numBytes > 0) { message = new String(buffer, 0, numBytes); } } catch (IOException e) { e.printStackTrace(); } } }
public void closeMessage() { isReceiveMsg = false; this.interrupt();
if (inputStream != null) { try { inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } if (outputStream != null) { try { outputStream.flush(); outputStream.close(); } catch (IOException e) { e.printStackTrace(); } } }
public void write(byte[] bytes) { try { outputStream.write(bytes); } catch (IOException e) { e.printStackTrace(); } } }
|
在添加直接写入和读取消息的方法,方便外部调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
|
public String getMessage() { String _message = message; message = null; return _message; }
public void sendMessage(String msg) { sendMessage(msg.getBytes()); }
public void sendMessage(byte[] bytes) { if (messageThread != null) { messageThread.write(bytes); } }
|
这样的话,在上面的建立蓝牙连接之后,就可以通过创建MessageThread对象来开启发送和接收消息,如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
|
private class ConnectThread extends Thread {
private final BluetoothSocket bluetoothSocket;
public ConnectThread(BluetoothSocket socket) { bluetoothSocket = socket; }
@Override public void run() { super.run(); cancelScanBluetoothDevices(); try { bluetoothSocket.connect(); messageThread = new MessageThread(bluetoothSocket); messageThread.start(); } catch (IOException e) { e.printStackTrace(); closeConnect(); } }
public void closeConnect() { if (bluetoothSocket != null) { try { bluetoothSocket.close(); } catch (IOException e) { e.printStackTrace(); } } if (messageThread != null) { messageThread.closeMessage(); messageThread = null; } } }
|
其他方法
其他经常用到的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
|
public List<BluetoothDevice> getBluetoothBondList() { Set<BluetoothDevice> bondedDevices = bluetoothAdapter.getBondedDevices(); return new ArrayList<>(bondedDevices); }
public BluetoothDevice getDeviceByAddress(String address) { return bluetoothAdapter.getRemoteDevice(address); }
|
添加一个over()
方法,用来结束一切
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
|
public void over(Context context) { cancelScanBluetoothDevices(); unregister(context); clearBluetoothDeviceList(); if (onScanBluetoothDeviceListener != null) { onScanBluetoothDeviceListener = null; } if (connectThread != null) { connectThread.closeConnect(); connectThread = null; } }
|
使用方式
在onCreate()
方法中,先注册接收蓝牙的通知
1
| OBDManager.getInstance().register(this);
|
在onResume()
方法中,使用initBlue()
方法初始化蓝牙
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
|
private void initBlue() { OBDManager obdManager = OBDManager.getInstance();
boolean hasBluetooth = obdManager.isHasBluetooth(this); if (hasBluetooth) { boolean isEnableBluetooth = obdManager.isEnableBluetooth(); if (isEnableBluetooth) { initList(); obdManager.startScanBluetoothDevices(bluetoothDevice -> { initList(); }); } else { boolean enableBluetooth = obdManager.enableBluetooth(); if (enableBluetooth) { handler.postDelayed(this::initBlue, 1000); } else { } } } else { } }
|
initBlue()
中调用了initList()
使搜索到的附近蓝牙设备在列表中显示,点击列表item时判断这个item所代表的蓝牙设备是否配对,如果已经配对则建立通信连接,如果没有配对则显示一个输入配对码的弹窗,输入配对码后点击确定进行配对,这里注意一下,OBD设备的配对码一般是“1234”或者“0000”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| private RecyclerView blue_list = findViewById(R.id.blue_list); private BlueAdapter blueAdapter; private DialogX dialogX;
private void initList() { OBDManager obdManager = OBDManager.getInstance();
if (blueAdapter != null) { blueAdapter.setData(obdManager.getBluetoothDeviceList()); } else { blueAdapter = new BlueAdapter(this, obdManager.getBluetoothDeviceList()); LinearLayoutManager layoutManager = new LinearLayoutManager(this, RecyclerView.VERTICAL, false); blue_list.setAdapter(blueAdapter); blue_list.setLayoutManager(layoutManager);
blueAdapter.setOnRecyclerItemClick((position, bluetoothDevice) -> { if (obdManager.isBondDevice(bluetoothDevice)) { obdManager.connectToDevice(bluetoothDevice); } else { dialogX = DialogXUtil.showEditTwoButtonDialog(this, "请输入配对码", "", "配对码", "确定", "取消", InputType.TYPE_CLASS_TEXT, true, new DialogXUtil.OnEditDialogOnSureButtonClick() { @Override public void ClickListener(EditText dialog_edit) { dialogX.dismiss(); String string = dialog_edit.getText().toString(); obdManager.bondDevice(bluetoothDevice, string); } }, new DialogXUtil.OnEditDialogOnCancelButtonClick() { @Override public void ClickListener(EditText dialog_edit) { dialogX.dismiss(); } }); } }); } }
|
再添加几个按钮,调用其他方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| open_blue.setOnClickListener(view -> { boolean enableBluetooth = OBDManager.getInstance().enableBluetooth(); if (enableBluetooth) { TickApplication.getInstance().speak("蓝牙启用成功"); } else { TickApplication.getInstance().speak("蓝牙启用失败"); } });
close_blue.setOnClickListener(view -> { boolean disableBluetooth = OBDManager.getInstance().disableBluetooth(); if (disableBluetooth) { TickApplication.getInstance().speak("蓝牙关闭成功"); } else { TickApplication.getInstance().speak("蓝牙关闭失败"); } });
notify_blue.setOnClickListener(view -> { OBDManager obdManager = OBDManager.getInstance(); obdManager.over(this); obdManager.register(this); initBlue(); });
|
最后,在onDestory()
方法中调用OBDManager
类下的over()
方法结束一切
1
| OBDManager.getInstance().over(this);
|
效果预览
列表中第三个那一堆东西是我在验证某个蓝牙设备已经匹配之后,显示它的所有UUID,打开设置是跳转到系统设置,缺点是没有在界面中添加通信功能,代码里都有
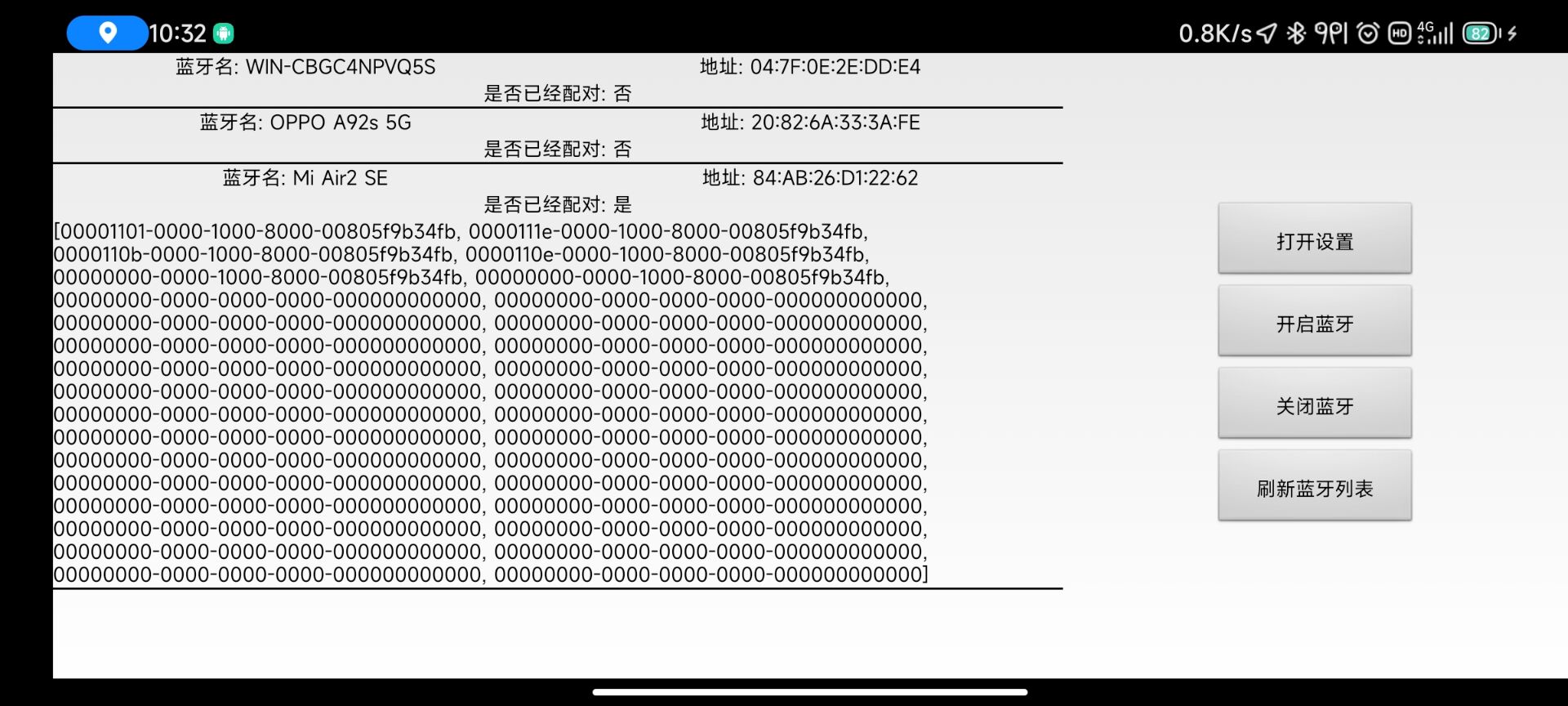
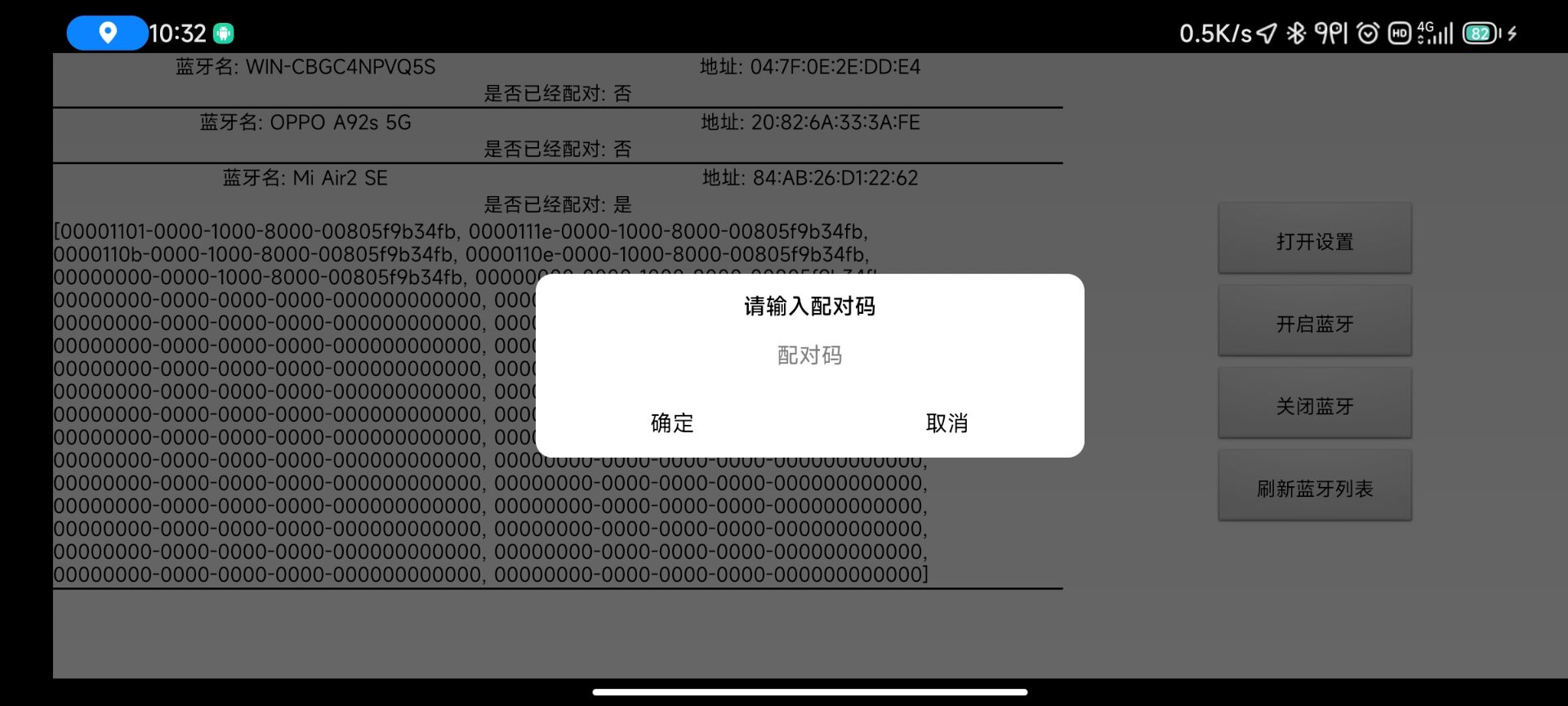